Java如何把文件夹打成压缩包并导出
时间:2023-03-21 09:47:34|栏目:JAVA代码|点击: 次
把文件夹打成压缩包并导出
1.打压缩包业务类
@Controller public class AdminController { private String filePath = AdminController.class.getResource("/").getPath().split("WEB-INF")[0]+ "upload/"; @RequestMapping(value = "export_zip.htm", method = {RequestMethod.GET, RequestMethod.POST }) public void zipwordDownAction(HttpServletRequest request,HttpServletResponse response) throws Exception { //打包文件的存放路径 ZipCompressorByAnt zc = new ZipCompressorByAnt(filePath+ "/file.zip"); //需要打包的文件路径 zc.compress(filePath+ "/file/"); String contentType = "application/octet-stream"; try { //导出压缩包 download(request, response, "upload/file.zip", contentType,encodeChineseDownloadFileName(request, "file.zip")); } catch (Exception e) { request.getSession().setAttribute("msg", "暂无内容"); } //如果原压缩包存在,则删除 File file=new File(filePath+ "/file.zip"); if(file.exists()){ file.delete(); } } /** * 下载文件 */ public static void download(HttpServletRequest request,HttpServletResponse response, String storeName, String contentType,String realName) throws Exception { response.setContentType("text/html;charset=UTF-8"); request.setCharacterEncoding("UTF-8"); BufferedInputStream bis = null; BufferedOutputStream bos = null; String ctxPath =FileUtil.class.getResource("/").getPath().split("WEB-INF")[0]; String downLoadPath = ctxPath + storeName; long fileLength = new File(downLoadPath).length(); response.setContentType(contentType); response.setHeader("Content-disposition", "attachment; filename=" + new String(realName.getBytes("utf-8"), "ISO8859-1")); response.setHeader("Content-Length", String.valueOf(fileLength)); bis = new BufferedInputStream(new FileInputStream(downLoadPath)); bos = new BufferedOutputStream(response.getOutputStream()); byte[] buff = new byte[2048]; int bytesRead; while (-1 != (bytesRead = bis.read(buff, 0, buff.length))) { bos.write(buff, 0, bytesRead); } bis.close(); bos.close(); } /** * 对文件流输出下载的中文文件名进行编码 屏蔽各种浏览器版本的差异性 */ public static String encodeChineseDownloadFileName(HttpServletRequest request, String pFileName) throws UnsupportedEncodingException { String filename = null; String agent = request.getHeader("USER-AGENT"); if (null != agent){ if (-1 != agent.indexOf("Firefox")) {//Firefox filename = "=?UTF-8?B?" + (new String(org.apache.commons.codec.binary.Base64.encodeBase64(pFileName.getBytes("UTF-8"))))+ "?="; }else if (-1 != agent.indexOf("Chrome")) {//Chrome filename = new String(pFileName.getBytes(), "ISO8859-1"); } else {//IE7+ filename = java.net.URLEncoder.encode(pFileName, "UTF-8"); filename = StringUtils.replace(filename, "+", "%20");//替换空格 } } else { filename = pFileName; } return filename; }
2.调用工具类
import java.io.File; import org.apache.tools.ant.Project; import org.apache.tools.ant.taskdefs.Zip; import org.apache.tools.ant.types.FileSet; public class ZipCompressorByAnt { private File zipFile; public ZipCompressorByAnt(String pathName) { zipFile = new File(pathName); } public void compress(String srcPathName) { File srcdir = new File(srcPathName); if (!srcdir.exists()) throw new RuntimeException(srcPathName + "不存在!"); Project prj = new Project(); Zip zip = new Zip(); zip.setProject(prj); zip.setDestFile(zipFile); FileSet fileSet = new FileSet(); fileSet.setProject(prj); fileSet.setDir(srcdir); //fileSet.setIncludes("**/*.java"); 包括哪些文件或文件夹 eg:zip.setIncludes("*.java"); //fileSet.setExcludes(...); 排除哪些文件或文件夹 zip.addFileset(fileSet); zip.execute(); } }
生成zip文件并导出
总结一下
关于Java下载zip文件并导出的方法,浏览器导出。
String downloadName = "下载文件名称.zip"; downloadName = BrowserCharCodeUtils.browserCharCodeFun(request, downloadName);//下载文件名乱码问题解决 //将文件进行打包下载 try { OutputStream out = response.getOutputStream(); byte[] data = createZip("/fileStorage/download");//服务器存储地址 response.reset(); response.setHeader("Content-Disposition","attachment;fileName="+downloadName); response.addHeader("Content-Length", ""+data.length); response.setContentType("application/octet-stream;charset=UTF-8"); IOUtils.write(data, out); out.flush(); out.close(); } catch (Exception e) { e.printStackTrace(); }
获取下载zip文件流
public byte[] createZip(String srcSource) throws Exception{ ByteArrayOutputStream outputStream = new ByteArrayOutputStream(); ZipOutputStream zip = new ZipOutputStream(outputStream); //将目标文件打包成zip导出 File file = new File(srcSource); a(zip,file,""); IOUtils.closeQuietly(zip); return outputStream.toByteArray(); }
public void a(ZipOutputStream zip, File file, String dir) throws Exception { //如果当前的是文件夹,则进行进一步处理 if (file.isDirectory()) { //得到文件列表信息 File[] files = file.listFiles(); //将文件夹添加到下一级打包目录 zip.putNextEntry(new ZipEntry(dir + "/")); dir = dir.length() == 0 ? "" : dir + "/"; //循环将文件夹中的文件打包 for (int i = 0; i < files.length; i++) { a(zip, files[i], dir + files[i].getName()); //递归处理 } } else { //当前的是文件,打包处理 //文件输入流 BufferedInputStream bis = new BufferedInputStream(new FileInputStream(file)); ZipEntry entry = new ZipEntry(dir); zip.putNextEntry(entry); zip.write(FileUtils.readFileToByteArray(file)); IOUtils.closeQuietly(bis); zip.flush(); zip.closeEntry(); } }
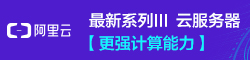
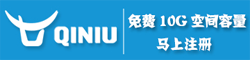