Java中DataInputStream和DataOutputStream的使用方法
简介
在 io 包中,提供了两个与平台无关的数据操作流:数据输出流(DataOutputStream)、数据输入流 (DataInputStream)。
通常数据输出流会按照一定的格式将数据输出,再通过数据输入流按照一定的格式将数据读入。DataOutputStream 和 DataOutputStream 用来读写固定字节格式数据。
DataOutputStream
创建对象
DataOutputStream out = new DataOutputStream(相接的流)
方法将一个 int 类型的数据写到数据输出流中,底层将 4 个字节写到基础输出流中
writeInt(int i)
将一个 double 类型的数据写到数据输出流中,底层会将 double 转换成 long 类型,写到基础输出流中,输出8个字节
writeDouble(double d)
以机器无关的方式使用 utf-8 编码方式将字符串写到基础输出流中。先输出 2 个字节表示字符串的字节长度,再输出这些字节值
writeUTF()
DataInputStream
创建对象
DataInputStream dis = new DataInputStream(InputStream in);
方法从数据输入流中读取一个 int 类型数据,读取 4 个字节
readInt()
读取8个字节
readDouble()
先读取 2 个字节来确定字符串的字节长度,再读取这些字节值
readUTF()
Tips:读取结束,再读取会出现EOFException
栗子1:写入数据
public class Main { public static void main(String[] args) throws Exception { DataOutputStream out = new DataOutputStream(new FileOutputStream("d:/abc/f5")); out.writeInt(20211011); out.writeUTF("晴,18度"); out.writeInt(20211012); out.writeUTF("晴,19度"); out.writeInt(20211013); out.writeUTF("多云,17度"); out.close(); } }
运行结果:
栗子2:读取
public class Main { public static void main(String[] args) throws Exception { DataInputStream in = new DataInputStream(new FileInputStream("d:/abc/f5")); try { while (true) { int date = in.readInt(); String s = in.readUTF(); System.out.println(date); System.out.println(s); } } catch (EOFException e) { //正确读取结束,不需要处理 } in.close(); } }
运行结果:
栗子3:保存学生信息
要求用如下格式保存学生信息:
学号 00 00 00 01
姓名 00 03 61 62 63
性别 00 61
年龄 00 00 00 16
xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:padding="20dp"> <EditText android:id="@+id/et1" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="学号" /> <EditText android:id="@+id/et2" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="姓名" /> <EditText android:id="@+id/et3" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="性别" /> <EditText android:id="@+id/et4" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="年龄" /> <Button android:id="@+id/btn1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="保存" /> <Button android:id="@+id/btn2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="读取" /> <TextView android:id="@+id/tv" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="10dp" /> </LinearLayout>
java
public class IoActivity extends AppCompatActivity { private EditText et1; private EditText et2; private EditText et3; private EditText et4; private Button btn1; private Button btn2; private TextView tv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_io); setViews(); setListeners(); } private void setViews() { et1 = findViewById(R.id.et1); et2 = findViewById(R.id.et2); et3 = findViewById(R.id.et3); et4 = findViewById(R.id.et4); btn1 = findViewById(R.id.btn1); btn2 = findViewById(R.id.btn2); tv = findViewById(R.id.tv); } private void setListeners() { btn1.setOnClickListener(view -> baocun()); btn2.setOnClickListener(view -> duqu()); } private void baocun() { //IO操作有IO异常,所以进行try...catch... /* * * ┌DataOutputStream * ┌FileOutputStream * sdcard */ try { int id = Integer.parseInt(et1.getText().toString()); String name = et2.getText().toString(); String gender = et3.getText().toString(); int age = Integer.parseInt(et4.getText().toString()); DataOutputStream out = new DataOutputStream( new FileOutputStream(getExternalFilesDir(null) + "/stu.txt", true) ); out.writeInt(id); out.writeUTF(name); out.writeChar(gender.charAt(0)); out.writeInt(age); out.close(); Toast.makeText(this, "保存成功", Toast.LENGTH_SHORT).show(); } catch (Exception e) { Toast.makeText(this, "保存失败", Toast.LENGTH_SHORT).show(); e.printStackTrace(); } } private void duqu() { //IO操作有IO异常,所以进行try...catch... try { DataInputStream in = new DataInputStream( new FileInputStream(getExternalFilesDir(null) + "/stu.txt") ); try { tv.setText(""); while (true) { int id = in.readInt(); String name = in.readUTF(); char gender = in.readChar(); int age = in.readInt(); tv.append("id:" + id + "\n" + "name:" + name + "\n" + "gender:" + gender + "\n" + "age:" + age + "\n"); } } catch (EOFException e) { } in.close(); Toast.makeText(this, "读取成功", Toast.LENGTH_SHORT).show(); } catch (Exception e) { Toast.makeText(this, "读取失败", Toast.LENGTH_SHORT).show(); e.printStackTrace(); } } }
运行程序:
点击读取按钮:
其中getExternalFilesDir(null)
得到以下路径
/storage/emulated/0/Android/data/yourPackageName/files
这个目录会在应用被卸载的时候删除,而且访问这个目录不需要动态申请STORAGE 权限。
所以运行程序会在这个路径下生成一个 stu.txt 的文件
上一篇:Spring boot2.0 实现日志集成的方法(3)
栏 目:JAVA代码
本文标题:Java中DataInputStream和DataOutputStream的使用方法
本文地址:http://www.codeinn.net/misctech/227806.html
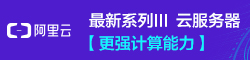
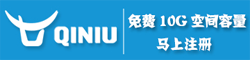