JavaScript获取对象key的几种方法和区别
1、Object.keys()遍历自身可以枚举属性
let myColors = { color1: 'pink', color2: 'red' }; let yourColors = { color3: 'green', color4: 'blue' }; Object.setPrototypeOf(yourColors, myColors); //setPrototypeOf()设置一个指定的对象的原型到另一个对象or NULL Object.keys(myColors); Object.keys(yourColors); console.log(myColors); console.log(yourColors); console.log(myColors['color1']); console.log(yourColors['color3']);
解析:Object.keys(myColors)
返回 myColors对象的自身可枚举属性键;Object.keys(yourColors)也是返回yourColors对象自身的可枚举属性键。setPrototypeOf()方法让yourColors继承myColors原型的属性,但是能看到并不能遍历出来。Object.keys() 是 遍历自身可以枚举属性。
2、Ojbect.values() /Ojject.entries()
返回自身可枚举属性的键值对数组:
let myColors = { color1: 'pink', color2: 'red' }; let yourColors = { color3: 'green', color4: 'blue' }; Object.setPrototypeOf(yourColors, myColors); console.log(Object.values(myColors)); console.log(Object.entries(myColors));
3、for-in 遍历可枚举属性prototype 属性
for-in遍历对象所有的可枚举属性,包括原型。
ps:for-in和for-of的区别
①for in 遍历的是数组的索引(即键名),for of遍历的是数组元素值
②for in 得到对象的key or 数组 or 字符串的下标
③for of 得到对象的value or 数组 or 字符串的值
for in更适合遍历对象
let myColors = { color1: 'pink', color2: 'red' }; let yourColors = { color3: 'green', color4: 'blue' }; Object.setPrototypeOf(yourColors, myColors); let arrayColors = []; for (let key in yourColors) { arrayColors.push(key); } console.log(arrayColors);
解析:arrayColors
数组 包含yourColors
自身属性键,也有从原型对象myColrs
继承的属性。
4、hasOwnProperty 遍历可枚举属性
返回一个布尔值,只能判断自有属性是否存在,对于继承属性会返回false
,因为它不查找原型链的函数
//不使用hasOwnProperty,返回自身属性和继承原型属性 let myColors = { color1: 'pink', color2: 'red' }; let yourColors = { color3: 'green', color4: 'blue' }; Object.setPrototypeOf(yourColors, myColors); for (let i in yourColors) { console.log(i); }
//使用hasOwnProperty,返回自身属性 let myColors = { color1: 'pink', color2: 'red' }; let yourColors = { color3: 'green', color4: 'blue' }; Object.setPrototypeOf(yourColors, myColors); for (let i in yourColors) { if (yourColors.hasOwnProperty(i)) { //加上if判断去掉原型链上的 console.log(i) } }
5、getOwnPropertyNames() 返回可枚举属性和不可枚举属性
不包括prototype属性,不包括symbol类型的key
getOwnPropertyNames()
返回一个对象自身所有的属性,包括可枚举和不可枚举属性组成的数组
//返回对象自身所有的属性,可枚举和不可枚举组成的数组,但不包括prototype属性 let myColors = { color1: 'pink', color2: 'red' }; let yourColors = { color3: 'green', color4: 'blue' }; Object.setPrototypeOf(yourColors, myColors); //定义不可枚举属性 Object.defineProperty(yourColors, 'your', { enumerable: true, value: 6, }) console.log(Object.getOwnPropertyNames(yourColors));
//返回对象自身所有的属性,可枚举和不可枚举组成的数组,但不包括Symbol类型的key let _item = Symbol('item') //定义Symbol数据类型 let myColors = { color1: 'pink', color2: 'red', }; let yourColors = { color3: 'green', color4: 'blue', [_item]: 'mySymbol' }; Object.setPrototypeOf(yourColors, myColors); //定义不可枚举属性 Object.defineProperty(yourColors, 'your', { enumerable: true, value: 6, }) console.log(Object.getOwnPropertyNames(yourColors));
6、getOwnPropertySymbols() 返回symbol类型的key属性,
不关心是否可枚举,返回对象自身的所有Symbol
属性组成的数组
let _item = Symbol('item') //定义Symbol数据类型 let myColors = { color1: 'pink', color2: 'red', }; let yourColors = { color3: 'green', color4: 'blue', [_item]: 'mySymbol' }; Object.setPrototypeOf(yourColors, myColors); //定义不可枚举属性 Object.defineProperty(yourColors, 'your', { enumerable: true, value: 6, }) console.log(Object.getOwnPropertySymbols(yourColors));
7、对象对key的获取方法
function getkey() { let obj = { a: 1, b: 2, c: 3 }; Object.prototype.d = 4; Object.defineProperty(obj, 'e', { configurable: true, writable: false, enumerable: false, value: 5 }); Object.defineProperty(obj, 'f', { configurable: true, writable: false, enumerable: true, value: 6 }); const symbolg = Symbol('g'); const symbolh = Symbol('h'); Object.defineProperty(obj, symbolg, { configurable: true, writable: false, enumerable: false, value: 7 }); Object.defineProperty(obj, symbolh, { configurable: true, writable: false, enumerable: true, value: 8 }); console.log(); for (let key in obj) { console.log('-- for-in:', key); if (obj.hasOwnProperty(key)) { console.log('-- hasOwnProperty: ', key); } } console.log('-- getOwnPropertyNames: ', Object.getOwnPropertyNames(obj)); console.log('-- getOwnPropertyDescriptor: ', Object.getOwnPropertyDescriptor(obj)); console.log('-- getOwnPropertySymbols: ', Object.getOwnPropertySymbols(obj)); console.log('-- keys: ', Object.keys(obj)); } /*** * -- for-in: a -- hasOwnProperty: a -- for-in: b -- hasOwnProperty: b -- for-in: c -- hasOwnProperty: c -- for-in: f -- hasOwnProperty: f -- for-in: d -- getOwnPropertyNames: (5) ["a", "b", "c", "e", "f"] -- getOwnPropertyDescriptor: undefined (可获取对象属性的具体配置,总共是6个) -- getOwnPropertySymbols: (2) [Symbol(g), Symbol(h)] -- keys: (4) ["a", "b", "c", "f"] */
8、对象声明/对象赋值
/** * 对象声明 * 首选 {} * * 对象赋值 * 首选 对象内赋值 */ function getKey(flag) { return `uniqued key ${flag}`; } const obj = { id: 5, name: 'San Francisco', [getKey('enabled')]: true, // 可变key提前声明 };
9、对象扩展
/** * * 对象 扩展 * * object assign 对象扩展 * 每次执行 assign * 事实上是对 object 原来对象的扩展然后并返回这个新的对象,? * 原理的对象被修改 * * */ const row = { display: 'inline-block', height: '50px', lineHeight: '50px', } const rowLeft = Object.assign(row, { color: 'rgba(0,0,0,.4)' }); const rowRight = Object.assign(row, { color: 'rgba(0,0,0,.6)' }); console.log(rowLeft, rowRight, '同时都被修改为最新的assign值');
上一篇:图片自动更新(说明)
栏 目:JavaScript代码
本文标题:JavaScript获取对象key的几种方法和区别
本文地址:http://www.codeinn.net/misctech/209121.html
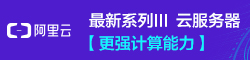
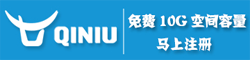