微信小程序连接MySQL数据库的全过程
时间:2022-07-17 09:14:59|栏目:JavaScript代码|点击: 次
简要说明:
承接上一个商品列表页,在服务器端连接MySQL数据库,通过条件匹配查寻数据并显示在客户端
准备工作
1、node.js
2、微信开发者工具
3、MySQL数据库
MySQL配置数据库、数据表
通过可视化的Workbench,可以很容易的建立自己的数据库、数据表。这里直接截个图就好了
推荐一个工具 Navicat for MySQL,以后可以通过它连接自己的数据库
目录结构
客户端代码实现
index.wxml (变化不大,加了跳转按钮)
<view class="contain"> <form bindsubmit="submit"> <view> <text id="top">商品</text> <text id="r" bindtap="jump">了解更多</text> <!-- <button type="default" bindtap="jump">了解更多</button> --> <!-- <button>详情</button> --> <checkbox-group name="skills"> <label wx:for="{{skill}}" wx:key="value"> <checkbox value="{{item.value}}" checked="{{item.checked}}"> <!-- {{item.name}} --> <image id="img" src="../image/{{item.name}}.jpg"></image> <view><text>{{item.text}}{{}}</text></view> </checkbox> </label> </checkbox-group> </view> <button form-type="submit">提交</button> <text id="sum">合计:{{result}}</text> </form> </view>
index.wxss
/* pages/index/index.wxss */ .contain{ /* background-color: aqua; */ margin: 15px auto; } #top{ /* margin:0 auto; */ margin-left: 20px; } #r{ margin-left: 150px; } #img{ /* float: left; */ width: 120px; height: 120px; } label{ height: 150px; position: relative; display: block; margin-left: 20px; } label view{ position: absolute; display: inline; padding-right: 20px; padding-top: 50px; } #sum{ margin-left: 20px; }
index.js (变化不大,加了跳转函数)
// pages/index/index.js Page({ /** * 页面的初始数据 */ data: { skill: [ {name:'01',value:600,checked:false,text:'宇智波佐助\n价格: 600.00'}, {name:'02',value:300,checked:false,text:'宇智波鼬\n价格: 300.00'}, {name:'03',value:500,checked:false,text:'旗木卡卡西\n价格: 500.00'}, {name:'04',value:700,checked:false,text:'路飞、红发香克斯\n价格: 700.00'}, {name:'07',value:350,checked:true,text:'索隆\n价格: 350.00'}, {name:'08',value:799,checked:true,text:'路飞\n价格: 799.00'}, ], result:[], names:[] }, /** * 生命周期函数--监听页面加载 */ onLoad: function (options) { var that =this wx.request({ url: 'http://127.0.0.1:3000/', success:function(res){ // console.log(res.data) that.setData({names:res.data}) } }) }, /** * 生命周期函数--监听页面初次渲染完成 */ onReady: function () { }, /** * 生命周期函数--监听页面显示 */ onShow: function () { }, /** * 生命周期函数--监听页面隐藏 */ onHide: function () { }, /** * 生命周期函数--监听页面卸载 */ onUnload: function () { }, /** * 页面相关事件处理函数--监听用户下拉动作 */ onPullDownRefresh: function () { }, /** * 页面上拉触底事件的处理函数 */ onReachBottom: function () { }, /** * 用户点击右上角分享 */ onShareAppMessage: function () { }, submit:function(e){ var that=this wx.request({ method:'POST', url: 'http://127.0.0.1:3000', data:e.detail.value, success:function (res){ const a=res.data.skills console.log(a) //求和计算 const reducer=(accumlator,currentValue)=>parseInt(accumlator)+parseInt(currentValue) console.log(a.reduce(reducer)) const sum=a.reduce(reducer) that.setData({result:sum}) } }) }, jump:function(){ wx.navigateTo({ url: '../about/about', }) } })
index.json (未做修改)
about.wxml
<!--pages/about/about.wxml--> <view> <view id="look"> <text>查看一下他们的详细资料吧!</text> </view> <form bindsubmit="submit"> <view class="select"> <input id="input" name="name" type="text" value="路飞"/> <button form-type="submit" id="btn">搜索</button> </view> </form> <view id="result"> <text>搜索结果:</text> <textarea name="" id="out" cols="30" rows="10">{{text[0].detail}}</textarea> </view> <button id="bottom" bindtap="back">返回</button> </view>
about.wxss
/* pages/about/about.wxss */ #look{ margin-top: 20px; margin-bottom: 20px; } #input{ border: 1px solid gray; } #btn{ margin-top: 10px; } #out{ border: 1px solid gray; } #bottom{ margin-top: 50px; } #result{ margin-top: 20px; }
about.js
// pages/about/about.js Page({ /** * 页面的初始数据 */ data: { text:{} }, /** * 生命周期函数--监听页面加载 */ onLoad: function (options) { }, /** * 生命周期函数--监听页面初次渲染完成 */ onReady: function () { }, /** * 生命周期函数--监听页面显示 */ onShow: function () { }, /** * 生命周期函数--监听页面隐藏 */ onHide: function () { }, /** * 生命周期函数--监听页面卸载 */ onUnload: function () { }, /** * 页面相关事件处理函数--监听用户下拉动作 */ onPullDownRefresh: function () { }, /** * 页面上拉触底事件的处理函数 */ onReachBottom: function () { }, /** * 用户点击右上角分享 */ onShareAppMessage: function () { }, back:function(){ wx.navigateBack() }, //提交 submit:function(e){ var that=this wx.request({ method:'POST', data:e.detail.value, url: 'http://127.0.0.1:3000/show', success:function(res){ // console.log(res.data) that.setData({text:res.data}) } }) } })
about.json
{ "navigationBarBackgroundColor":"#fff", "navigationBarTitleText":"详情", "navigationBarTextStyle":"black", "usingComponents": {} }
服务器端代码实现
server.js
const express=require('express') const bodyParser =require('body-parser') const app=express() const mysql = require('mysql') app.use(bodyParser.json()) //处理post请求 app.post('/',(req,res) => { console.log(req.body) res.json(req.body) }) app.post('/show',(req,res)=>{ console.log(req.body.name) const a=req.body.name var connection=mysql.createConnection({ host:'localhost', user:'你的用户名', password:'你的密码', database:'数据库名字' }) connection.connect(); connection.query("select detail from price where name='"+a+"'",function(error,results,fields){ if(error) throw console.error; res.json(results) console.log(results) }) connection.end(); }) app.get('/',(req,res)=>{ var connection = mysql.createConnection({ host:'localhost', user:'你的用户名', password:'你的密码', database:'数据库名字' }); connection.connect(); //查找所有的人物名字返回给客户端。其实没必要(测试用的) connection.query('select name from price',function(error,results,fields){ if(error) throw error; res.json(results) // console.log(results) }) connection.end(); }) app.listen(3000,()=>{ console.log('server running at http://127.0.0.1:3000') })
效果展示
主界面
跳转界面
界面有点丑,慢慢优化
总结
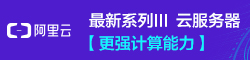
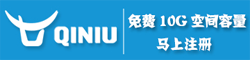