Android LayoutInflater.inflate源码分析
LayoutInflater.inflate源码详解
LayoutInflater的inflate方法相信大家都不陌生,在Fragment的onCreateView中或者在BaseAdapter的getView方法中我们都会经常用这个方法来实例化出我们需要的View.
假设我们有一个需要实例化的布局文件menu_item.xml:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <TextView android:id="@+id/id_menu_title_tv" android:layout_width="match_parent" android:layout_height="300dp" android:gravity="center_vertical" android:textColor="@android:color/black" android:textSize="16sp" android:text="@string/menu_item"/> </LinearLayout>
我们想在BaseAdapter的getView()方法中对其进行实例化,其实例化的方法有三种,分别是:
2个参数的方法:
convertView = mInflater.inflate(R.layout.menu_item, null);
3个参数的方法(attachToRoot=false):
convertView = mInflater.inflate(R.layout.menu_item, parent, false);
3个参数的方法(attachToRoot=true):
convertView = mInflater.inflate(R.layout.menu_item, parent, true);
究竟我们应该用哪个方法进行实例化View,这3个方法又有什么区别呢?如果有同学对三个方法的区别还不是特别清楚,那么就和我一起从源码的角度来分析一下这个问题吧.
源码
inflate
我们先来看一下两个参数的inflate方法,源码如下:
public View inflate(@LayoutRes int resource, @Nullable ViewGroup root) { return inflate(resource, root, root != null); }
从代码我们看出,其实两个参数的inflate方法根据父布局parent是否为null作为第三个参数来调用三个参数的inflate方法,三个参数的inflate方法源码如下:
public View inflate(@LayoutRes int resource, @Nullable ViewGroup root, boolean attachToRoot) { // 获取当前应用的资源集合 final Resources res = getContext().getResources(); // 获取指定资源的xml解析器 final XmlResourceParser parser = res.getLayout(resource); try { return inflate(parser, root, attachToRoot); } finally { // 返回View之前关闭parser资源 parser.close(); } }
这里需要解释一下,我们传入的资源布局id是无法直接实例化的,需要借助XmlResourceParser.
而XmlResourceParser是借助Android的pull解析方法是解析布局文件的.继续跟踪inflate方法源码:
public View inflate(XmlPullParser parser, @Nullable ViewGroup root, boolean attachToRoot) { synchronized (mConstructorArgs) { // 获取上下文对象,即LayoutInflater.from传入的Context. final Context inflaterContext = mContext; // 根据parser构建XmlPullAttributes. final AttributeSet attrs = Xml.asAttributeSet(parser); // 保存之前的Context对象. Context lastContext = (Context) mConstructorArgs[0]; // 赋值为传入的Context对象. mConstructorArgs[0] = inflaterContext; // 注意,默认返回的是父布局root. View result = root; try { // 查找xml的开始标签. int type; while ((type = parser.next()) != XmlPullParser.START_TAG && type != XmlPullParser.END_DOCUMENT) { // Empty } // 如果没有找到有效的开始标签,则抛出InflateException异常. if (type != XmlPullParser.START_TAG) { throw new InflateException(parser.getPositionDescription() + ": No start tag found!"); } // 获取控件名称. final String name = parser.getName(); // 特殊处理merge标签 if (TAG_MERGE.equals(name)) { if (root == null || !attachToRoot) { throw new InflateException("<merge /> can be used only with a valid " + "ViewGroup root and attachToRoot=true"); } rInflate(parser, root, inflaterContext, attrs, false); } else { // 实例化我们传入的资源布局的view final View temp = createViewFromTag(root, name, inflaterContext, attrs); ViewGroup.LayoutParams params = null; // 如果传入的parent不为空. if (root != null) { if (DEBUG) { System.out.println("Creating params from root: " + root); } // 创建父类型的LayoutParams参数. params = root.generateLayoutParams(attrs); if (!attachToRoot) { // 如果实例化的View不需要添加到父布局上,则直接将根据父布局生成的params参数设置 // 给它即可. temp.setLayoutParams(params); } } // 递归的创建当前布局的所有控件 rInflateChildren(parser, temp, attrs, true); // 如果传入的父布局不为null,且attachToRoot为true,则将实例化的View加入到父布局root中 if (root != null && attachToRoot) { root.addView(temp, params); } // 如果父布局为null或者attachToRoot为false,则将返回值设置成我们实例化的View if (root == null || !attachToRoot) { result = temp; } } } catch (XmlPullParserException e) { InflateException ex = new InflateException(e.getMessage()); ex.initCause(e); throw ex; } catch (Exception e) { InflateException ex = new InflateException( parser.getPositionDescription() + ": " + e.getMessage()); ex.initCause(e); throw ex; } finally { // Don't retain static reference on context. mConstructorArgs[0] = lastContext; mConstructorArgs[1] = null; } Trace.traceEnd(Trace.TRACE_TAG_VIEW); return result; } }
上述代码中的关键部分我已经加入了中文注释.从上述代码中我们还可以发现,我们传入的布局文件是通过createViewFromTag来实例化每一个子节点的.
createViewFromTag
函数源码如下:
/** * 方便调用5个参数的方法,ignoreThemeAttr的值为false. */ private View createViewFromTag(View parent, String name, Context context, AttributeSet attrs) { return createViewFromTag(parent, name, context, attrs, false); } View createViewFromTag(View parent, String name, Context context, AttributeSet attrs, boolean ignoreThemeAttr) { if (name.equals("view")) { name = attrs.getAttributeValue(null, "class"); } // Apply a theme wrapper, if allowed and one is specified. if (!ignoreThemeAttr) { final TypedArray ta = context.obtainStyledAttributes(attrs, ATTRS_THEME); final int themeResId = ta.getResourceId(0, 0); if (themeResId != 0) { context = new ContextThemeWrapper(context, themeResId); } ta.recycle(); } // 特殊处理“1995”这个标签(ps: 平时我们写xml布局文件时基本没有使用过). if (name.equals(TAG_1995)) { // Let's party like it's 1995! return new BlinkLayout(context, attrs); } try { View view; if (mFactory2 != null) { view = mFactory2.onCreateView(parent, name, context, attrs); } else if (mFactory != null) { view = mFactory.onCreateView(name, context, attrs); } else { view = null; } if (view == null && mPrivateFactory != null) { view = mPrivateFactory.onCreateView(parent, name, context, attrs); } if (view == null) { final Object lastContext = mConstructorArgs[0]; mConstructorArgs[0] = context; try { if (-1 == name.indexOf('.')) { view = onCreateView(parent, name, attrs); } else { view = createView(name, null, attrs); } } finally { mConstructorArgs[0] = lastContext; } } return view; } catch (InflateException e) { throw e; } catch (ClassNotFoundException e) { final InflateException ie = new InflateException(attrs.getPositionDescription() + ": Error inflating class " + name); ie.initCause(e); throw ie; } catch (Exception e) { final InflateException ie = new InflateException(attrs.getPositionDescription() + ": Error inflating class " + name); ie.initCause(e); throw ie; } }
在createViewFromTag方法中,最终是通过createView方法利用反射来实例化view控件的.
createView
public final View createView(String name, String prefix, AttributeSet attrs) throws ClassNotFoundException, InflateException { // 以View的name为key, 查询构造函数的缓存map中是否已经存在该View的构造函数. Constructor<? extends View> constructor = sConstructorMap.get(name); Class<? extends View> clazz = null; try { // 构造函数在缓存中未命中 if (constructor == null) { // 通过类名去加载控件的字节码 clazz = mContext.getClassLoader().loadClass(prefix != null ? (prefix + name) : name).asSubClass(View.class); // 如果有自定义的过滤器并且加载到字节码,则通过过滤器判断是否允许加载该View if (mFilter != null && clazz != null) { boolean allowed = mFilter.onLoadClass(clazz); if (!allowed) { failNotAllowed(name, prefix, attrs); } } // 得到构造函数 constructor = clazz.getConstructor(mConstructorSignature); constructor.setAccessible(true); // 缓存构造函数 sConstructorMap.put(name, constructor); } else { if (mFilter != null) { // 过滤的map是否包含了此类名 Boolean allowedState = mFilterMap.get(name); if (allowedState == null) { // 重新加载类的字节码 clazz = mContext.getClassLoader().loadClass(prefix != null ? (prefix + name) : name).asSubclass(View.class); boolean allowed = clazz != null && mFilter.onLoadClass(clazz); mFilterMap.put(name, allowed); if (!allowed) { failNotAllowed(name, prefix, attrs); } } else if (allowedState.equals(Boolean.FALSE)) { failNotAllowed(name, prefix, attrs); } } } // 实例化类的参数数组(mConstructorArgs[0]为Context, [1]为View的属性) Object[] args = mConstructorArgs; args[1] = attrs; // 通过构造函数实例化View final View view = constructor.newInstance(args); if (View instanceof ViewStub) { final ViewStub viewStub = (ViewStub) view; viewStub.setLayoutInflater(cloneInContext((Context)args[0])) } return view; } catch (NoSunchMethodException e) { // ...... } catch (ClassNotFoundException e) { // ...... } catch (Exception e) { // ...... } finally { // ...... } }
总结
通过学习了inflate函数源码,我们再回过头去看BaseAdapter的那三种方法,我们可以得出的结论是:
第一种方法使用不够规范, 且会导致实例化View的LayoutParams属性失效.(ps: 即layout_width和layout_height等参数失效, 因为源码中这种情况的LayoutParams为null).
第二种是最正确,也是最标准的写法.
第三种由于attachToRoot为true,所以返回的View其实是父布局ListView,这显然不是我们想要实例化的View.因此,第三种写法是错误的.
感谢阅读,希望能帮助到大家,谢谢大家对本站的支持!
栏 目:Android代码
本文标题:Android LayoutInflater.inflate源码分析
本文地址:http://www.codeinn.net/misctech/198296.html
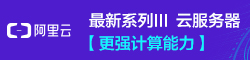
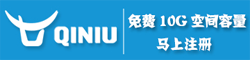