使用Python测试Ping主机IP和某端口是否开放的实例
时间:2020-11-06 10:18:47|栏目:Python代码|点击: 次
使用Python方法
比用各种命令方便,可以设置超时时间,到底通不通,端口是否开放一眼能看出来。
命令和返回
完整权限,可以ping通,端口开放,结果如下:
无root权限(省略了ping),端口开放,结果如下:
完整权限,可以ping通,远端端口关闭,结果如下:
完整权限,可以ping通,本地端口关闭,结果如下:
完整权限,不能ping通(端口自然也无法访问),结果如下:
pnp.py代码
#!/usr/bin/python #name pnp.py #ping and port #coding:utf-8 import os, sys, socket, struct, select, time ICMP_ECHO_REQUEST = 8 # Seems to be the same on Solaris. socket.setdefaulttimeout(4) #first argument host=sys.argv[1] #second argument port=int(sys.argv[2]) #socket try connect def PortOpen(ip,port): print( '\033[1m*Port\033[0m %s:%d' %(ip,port)), s=socket.socket(socket.AF_INET,socket.SOCK_STREAM) try: s.connect((ip,port)) s.shutdown(2) print( '\033[1;32m.... is OK.\033[0m' ) return True except socket.timeout: print( '\033[1;33m.... is down or network time out!!!\033[0m' ) return False except: print( '\033[1;31m.... is down!!!\033[0m' ) return False def checksum(source_string): """ I'm not too confident that this is right but testing seems to suggest that it gives the same answers as in_cksum in ping.c """ sum = 0 countTo = (len(source_string)/2)*2 count = 0 while count<countTo: thisVal = ord(source_string[count + 1])*256 + ord(source_string[count]) sum = sum + thisVal sum = sum & 0xffffffff # Necessary? count = count + 2 if countTo<len(source_string): sum = sum + ord(source_string[len(source_string) - 1]) sum = sum & 0xffffffff # Necessary? sum = (sum >> 16) + (sum & 0xffff) sum = sum + (sum >> 16) answer = ~sum answer = answer & 0xffff # Swap bytes. Bugger me if I know why. answer = answer >> 8 | (answer << 8 & 0xff00) return answer def receive_one_ping(my_socket, ID, timeout): """ receive the ping from the socket. """ timeLeft = timeout while True: startedSelect = time.time() whatReady = select.select([my_socket], [], [], timeLeft) howLongInSelect = (time.time() - startedSelect) if whatReady[0] == []: # Timeout return timeReceived = time.time() recPacket, addr = my_socket.recvfrom(1024) icmpHeader = recPacket[20:28] type, code, checksum, packetID, sequence = struct.unpack( "bbHHh", icmpHeader ) if packetID == ID: bytesInDouble = struct.calcsize("d") timeSent = struct.unpack("d", recPacket[28:28 + bytesInDouble])[0] return timeReceived - timeSent timeLeft = timeLeft - howLongInSelect if timeLeft <= 0: return def send_one_ping(my_socket, dest_addr, ID): """ Send one ping to the given >dest_addr<. """ dest_addr = socket.gethostbyname(dest_addr) # Header is type (8), code (8), checksum (16), id (16), sequence (16) my_checksum = 0 # Make a dummy heder with a 0 checksum. header = struct.pack("bbHHh", ICMP_ECHO_REQUEST, 0, my_checksum, ID, 1) #a1 = struct.unpack("bbHHh",header) #my test bytesInDouble = struct.calcsize("d") data = (192 - bytesInDouble) * "Q" data = struct.pack("d", time.time()) + data # Calculate the checksum on the data and the dummy header. my_checksum = checksum(header + data) # Now that we have the right checksum, we put that in. It's just easier # to make up a new header than to stuff it into the dummy. header = struct.pack("bbHHh", ICMP_ECHO_REQUEST, 0, socket.htons(my_checksum), ID, 1) packet = header + data my_socket.sendto(packet, (dest_addr, 1)) # Don't know about the 1 def do_one(dest_addr, timeout): """ Returns either the delay (in seconds) or none on timeout. """ delay=None icmp = socket.getprotobyname("icmp") try: my_socket = socket.socket(socket.AF_INET, socket.SOCK_RAW, icmp) my_ID = os.getpid() & 0xFFFF send_one_ping(my_socket, dest_addr, my_ID) delay = receive_one_ping(my_socket, my_ID, timeout) my_socket.close() except socket.error, (errno, msg): if errno == 1: # Operation not permitted msg = msg + ( " - not root." ) raise socket.error(msg) #raise # raise the original error return delay def verbose_ping(dest_addr, timeout = 2, count = 100): """ Send >count< ping to >dest_addr< with the given >timeout< and display the result. """ for i in xrange(count): print "\033[1m*Ping\033[0m %s ..." % dest_addr, try: delay = do_one(dest_addr, timeout) except socket.error, e: print "\033[1;31m... failed. (%s)" % e break if delay == None: print "\033[1;31m... failed. (timeout within %ssec.)\033[0m" % timeout else: delay = delay * 1000 print "\033[1;32m... get ping in %0.4fms\033[0m" % delay if __name__ == '__main__': if os.geteuid() == 0: verbose_ping(host,2,3) else: print "\033[1m*Ping\033[0m test must be sudo or root..." PortOpen(host,port) print( 'Job finished.')
使用命令方法
使用命令ping就不说了,端口可以用下面的命令。
当时目前telnet基本不用,可能没有telnet客户端了。
测试通常连接不上会等很久,端口连上了也需要通过反馈内容自行判断。
telnet
telnet ip port
$telnet 192.168.234.1 Trying 192.168.234.1... Connected to 192.168.234.1. Escape character is '^]'. ......
wget
wget ip:port
$wget 192.168.234.1:21 --2019-03-22 15:42:27-- http://192.168.234.1:21/ 正在连接 192.168.234.1:21... 已连接。 已发出 HTTP 请求,正在等待回应... 200 没有 HTTP 头,尝试 HTTP/0.9 长度:未指定 正在保存至: “index.html” ......
SSH
ssh -v ip -p port
$ssh -v 192.168.234.1 -p 21 OpenSSH_7.4p1, OpenSSL 1.0.2k-fips 26 Jan 2017 debug1: Reading configuration data /etc/ssh/ssh_config debug1: /etc/ssh/ssh_config line 58: Applying options for * debug1: Connecting to 192.168.234.1 [192.168.234.1] port 21. debug1: Connection established. ......
curl
culr ip:port
$curl 192.168.234.1:21 220 Serv-U FTP Server v15.1 ready... 530 Not logged in. ......
栏 目:Python代码
下一篇:Python multiprocess pool模块报错pickling error问题解决方法分析
本文标题:使用Python测试Ping主机IP和某端口是否开放的实例
本文地址:http://www.codeinn.net/misctech/19696.html
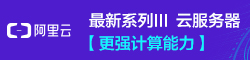
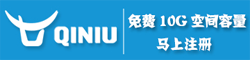